Take this c program that does nothing but exit.
#include<stdlib.h>
void main(){
exit(0);
}
Let's say you run this command to build...
gcc -S -masm=intel -static exit.c
Then if you view your directory you'll see you have an assembly file (exit.s)...
ls -l
-rw-rw-r-- 1 user user 140 May 20 12:02 exit.c
-rw-rw-r-- 1 user user 391 May 20 12:33 exit.s
Here is the output of the assembly file.
.file "exit.c"
.intel_syntax noprefix
.text
.globl main
.type main, @function
main:
.LFB2:
.cfi_startproc
push ebp
.cfi_def_cfa_offset 8
.cfi_offset 5, -8
mov ebp, esp
.cfi_def_cfa_register 5
and esp, -16
sub esp, 16
mov DWORD PTR [esp], 0
call exit
.cfi_endproc
.LFE2:
.size main, .-main
.ident "GCC: (Ubuntu xxxxx) xxxxx"
.section .note.GNU-stack,"",@progbits
The .file tells assembly that we're starting a new file with this name.
The .intel_syntax noprefix tells assembly we're using intel version, and we are not going to prefix registers with a % symbol
The .text tells assembly that the code section is now starting.
The .globl main tells assembly that main is a symbol that can be used outside this file. That is needed for the operating system to make the initial call to start this program.
The .type main, @function is used in conjunction with the previous directive to tell the outside linker that main is a function
The main: creates a label called main, which is what we just defined in the previous 2 directives as an externally accessible function
The LFB2: is an auto-generated label by the compiler that likely stands for LABEL FUNCTION BEGIN #2, and is generally seen prior to every function.
The .cfi_starproc is an auto-generated directive that the assembler will use during error handling to unwind an the stack.
The push ebp is setting up for your function call by saving the Base Pointer on the stack so you could restore it at the end of the function (of course this isn't used/necessary in this instance since we'll never return from this function as it's calling the exit syscall).
The .cfi_def_cfa_offset 8 is an auto-generated directive that the assembler will use during error handling to unwind an the stack.
The .cfi_offset 5, -8 is an auto-generated directive that the assembler will use during error handling to unwind an the stack.
The mov ebp, esp is also setting up for your function call by setting the new Base Pointer to the old Stack Pointer (basically saving off the previous stack for later use and starting a new stack for this scope).
The .cfi_def_cfa_register 5 is an auto-generated directive that the assembler will use during error handling to unwind an the stack.
The and esp, -16 is essentially the same as and esp, 11111111 11111111 11111111 11110000. Removing last 4 bits means rounding down to 16 byte boundary.
The sub esp, 16 is allocating 16 bytes for local variables.
The mov DWORD PTR [esp], 0 is saving the value 0 to the stack (basically passing 0 as a parameter to the next function).
The call exit is the call to the syscall that exits with return code 0 (our parameter) to the operating system.
The .cfi_endproc is an auto-generated directive that the assembler will use during error handling to unwind an the stack.
The LFE2: is an auto-generated label by the compiler that likely stands for LABEL FUNCTION END #2, and is generally seen at the end of every function.
The .size main, .-main tells the assembler to compute the size of symbol main to be the difference between the label main and the current position in the file
The .ident "GCC: (Ubuntu xxxxx) xxxxx" identifies which version of the compiler generated this assembly.
The .section .note.GNU-stack,"",@progbits is where gcc (the compiler we used) specific stack options are specified.
Copyright © 2015, this post cannot be reproduced or retransmitted in any form without reference to the original post.
Saturday, May 23, 2015
Wednesday, May 20, 2015
gcc static linking
Take this c program that does nothing but exit.
#include<stdlib.h>
void main(){
exit(0);
}
There is a gcc option to complile with '-static'.
Let's say you run this command to build...
gcc -o exit exit.c
Then if you view your directory you'll see you have an executable and code...
ls -l
-rwxrwxr-x 1 user user 7291 May 20 12:02 exit
-rw-rw-r-- 1 user user 140 May 20 12:02 exit.c
Let's say you run this command to build...
gcc -static -o exit exit.c
Then if you view your directory you'll see you have an executable and code...
ls -l
-rwxrwxr-x 1 user user 733094 May 20 12:02 exit
-rw-rw-r-- 1 user user 140 May 20 12:02 exit.c
First, let's noticed what I colored in green and red. The file sizes. Notice that the regular (dynamically linked) executable is relatively small. Notice that the statically linked (-static) executable though is quite large (a magnitude of 10x larger!). Why?
Because by statically linking the libraries, what we've told the compiler (gcc) to do is the take a copy of all libraries that are needed (such as stdlib.h), make a copy of them, and bundle them directly into the executable. If the libraries are dynamically linked, those libraries are not included in the executable, but instead are referenced/called from wherever they live on the device.
A con of static linking is that it can obviously cause disk space issues, since there is no library re-uses, instead every application built statically would have it's own independent copy of everything and it's eat up disk space.
Another con of static linking is that it can also cause compatibility issues because many times libraries are built to be machine dependent. If you statically built your executable, you only included the version of the library from which the executable was compiled on. Some of those libraries will then break on different machines.
But a pro of static linking is that it is the ideal deploy process for an application. There are no pre-requisites, no dependencies on library versions, etc., if it's on the right type of machine, it'll just work.
But the most interesting topic, and the reason for writing this blog post is Security. You should hopefully have put all the puzzle pieces together by now, and started to realize that static linking is BAD in terms of Security Vulnerabilities. Why? If an application is using a library, and that library contains a security vulnerabilities (buffer overflow, sql injection, etc.) ...
- Which do you think is going to be easier to patch? My Opinion: Dynamic is easier to patch because you just need to update the library (smaller set of code, easier to deploy/update, smaller subset to test, etc.)
- Which do you think is better for shared libraries? My Opinion: If a library is shared by multiple applications (like stdlib.h), and that library needs patched, it's much simpler to patch that one library than it would be to identify, patch, and redeploy every application using that library.
- Which do you think is more likely to expose old/missed security vulnerabilities? My Opinion: If a library has a vulnerability, it's much better to be able to patch it in 1 spot and have all applications fixed. If you have everything statically linked, you must remember which applications use that library, and make sure you patch and re-deploy each of them. What if you miss one application? Now you have an old unpatched vulnerability sitting out.
For that main reason, to my knowledge statically linked libraries are pretty much useless and should be avoided primarily because of the security risks they present.
Copyright © 2015, this post cannot be reproduced or retransmitted in any form without reference to the original post.
#include<stdlib.h>
void main(){
exit(0);
}
There is a gcc option to complile with '-static'.
Let's say you run this command to build...
gcc -o exit exit.c
Then if you view your directory you'll see you have an executable and code...
ls -l
-rwxrwxr-x 1 user user 7291 May 20 12:02 exit
-rw-rw-r-- 1 user user 140 May 20 12:02 exit.c
Let's say you run this command to build...
gcc -static -o exit exit.c
Then if you view your directory you'll see you have an executable and code...
ls -l
-rwxrwxr-x 1 user user 733094 May 20 12:02 exit
-rw-rw-r-- 1 user user 140 May 20 12:02 exit.c
First, let's noticed what I colored in green and red. The file sizes. Notice that the regular (dynamically linked) executable is relatively small. Notice that the statically linked (-static) executable though is quite large (a magnitude of 10x larger!). Why?
Because by statically linking the libraries, what we've told the compiler (gcc) to do is the take a copy of all libraries that are needed (such as stdlib.h), make a copy of them, and bundle them directly into the executable. If the libraries are dynamically linked, those libraries are not included in the executable, but instead are referenced/called from wherever they live on the device.
A con of static linking is that it can obviously cause disk space issues, since there is no library re-uses, instead every application built statically would have it's own independent copy of everything and it's eat up disk space.
Another con of static linking is that it can also cause compatibility issues because many times libraries are built to be machine dependent. If you statically built your executable, you only included the version of the library from which the executable was compiled on. Some of those libraries will then break on different machines.
But a pro of static linking is that it is the ideal deploy process for an application. There are no pre-requisites, no dependencies on library versions, etc., if it's on the right type of machine, it'll just work.
But the most interesting topic, and the reason for writing this blog post is Security. You should hopefully have put all the puzzle pieces together by now, and started to realize that static linking is BAD in terms of Security Vulnerabilities. Why? If an application is using a library, and that library contains a security vulnerabilities (buffer overflow, sql injection, etc.) ...
- Which do you think is going to be easier to patch? My Opinion: Dynamic is easier to patch because you just need to update the library (smaller set of code, easier to deploy/update, smaller subset to test, etc.)
- Which do you think is better for shared libraries? My Opinion: If a library is shared by multiple applications (like stdlib.h), and that library needs patched, it's much simpler to patch that one library than it would be to identify, patch, and redeploy every application using that library.
- Which do you think is more likely to expose old/missed security vulnerabilities? My Opinion: If a library has a vulnerability, it's much better to be able to patch it in 1 spot and have all applications fixed. If you have everything statically linked, you must remember which applications use that library, and make sure you patch and re-deploy each of them. What if you miss one application? Now you have an old unpatched vulnerability sitting out.
For that main reason, to my knowledge statically linked libraries are pretty much useless and should be avoided primarily because of the security risks they present.
Copyright © 2015, this post cannot be reproduced or retransmitted in any form without reference to the original post.
Friday, May 8, 2015
Analyze Internet History of IE Firefox Chrome and Safari
This BrowsingHistoryView tool seems pretty useful to analyze Internet Explorer, Mozilla Firefox, Google Chrome, and Safari browsing history.
Run the tool
>BrowsingHistoryView.exe /HistorySourceFolder 2
Select All, Copy
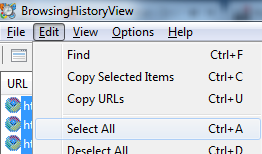
Paste into Excel
View the History in a nicer readable format
Copyright © 2015, this post cannot be reproduced or retransmitted in any form without reference to the original post.
Run the tool
>BrowsingHistoryView.exe /HistorySourceFolder 2
Select All, Copy
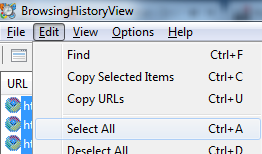
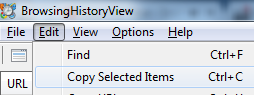
Paste into Excel
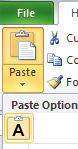
View the History in a nicer readable format
Copyright © 2015, this post cannot be reproduced or retransmitted in any form without reference to the original post.
McAfee Cookie Analyzer - Galleta
McAfee has a free tool called Galetta which seems to make viewing Internet Explorer Cookies a little easier.
1.) Find a cookie text file (in a location like C:\Users\XXXX\AppData\Roaming\Microsoft\Windows\Cookies\Low )
2.) Run Galleta
] galetta.exe C:\Users\XXXX\AppData\Roaming\Microsoft\Windows\Cookies\Low\EKHU3P50.txt > c:\cookies.txt
3.) Open the output cookies.txt with Excel
4.) View the cookies in a more readable format
Copyright © 2015, this post cannot be reproduced or retransmitted in any form without reference to the original post.
1.) Find a cookie text file (in a location like C:\Users\XXXX\AppData\Roaming\Microsoft\Windows\Cookies\Low )
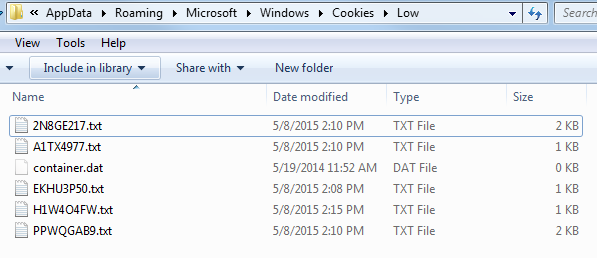
2.) Run Galleta
] galetta.exe C:\Users\XXXX\AppData\Roaming\Microsoft\Windows\Cookies\Low\EKHU3P50.txt > c:\cookies.txt
3.) Open the output cookies.txt with Excel

4.) View the cookies in a more readable format

Copyright © 2015, this post cannot be reproduced or retransmitted in any form without reference to the original post.
Thursday, May 7, 2015
Majority of Internet Encrypted by 2016
Couple of good articles around moving the Internet to HTTPS.
Because of NetFlix, more than half of the world’s Internet traffic will likely be encrypted by year end, says a report released by the Canadian networking equipment company Sandvine. The current share of encrypted traffic on the web is largely due to Google , Facebook , and Twitter , which have all by now adopted HTTPS by default.
Google said that the Github DDoS attack would not have been possible if the web had embraced moves to encrypt its transport layers. "This provides further motivation for transitioning the web to encrypted and integrity-protected communication," Google security engineer Niels Provos.
Firefox Web browser wants to see Web site encryption become standard practice. Mozilla said it plans to set a date by which all new features for its browser will be available only to secure Web sites. Additionally, it will gradually phase out access to some browser features for Web sites that continue to use HTTP instead of the certificate-secured HTTPS.
Copyright © 2015, this post cannot be reproduced or retransmitted in any form without reference to the original post.
Because of NetFlix, more than half of the world’s Internet traffic will likely be encrypted by year end, says a report released by the Canadian networking equipment company Sandvine. The current share of encrypted traffic on the web is largely due to Google , Facebook , and Twitter , which have all by now adopted HTTPS by default.
Google said that the Github DDoS attack would not have been possible if the web had embraced moves to encrypt its transport layers. "This provides further motivation for transitioning the web to encrypted and integrity-protected communication," Google security engineer Niels Provos.
Firefox Web browser wants to see Web site encryption become standard practice. Mozilla said it plans to set a date by which all new features for its browser will be available only to secure Web sites. Additionally, it will gradually phase out access to some browser features for Web sites that continue to use HTTP instead of the certificate-secured HTTPS.
Copyright © 2015, this post cannot be reproduced or retransmitted in any form without reference to the original post.
Subscribe to:
Posts (Atom)